require "erb"
include ERB::Util
title, description, url, topic = ARGV[0], ARGV[1], ARGV[2], ARGV[3]
puts <<EOS
<a href="http://adigg.com/submit?phase=2
&url=#{url_encode(url)}
&title=#{url_encode(title)}
&bodytext=#{url_encode(description)}
&topic=#{url_encode(topic)}">
<img src="http://digg.com/img/badges/91x17-digg-button.gif"
width="91" height="17" alt="Digg!" border="0" />
</a>
EOS
That worked reasonably well, but then I started thinking back to all the stuff I learned about Mac OS X software development with Cocoa and decided that I should really wrap up my cute little Ruby script with a Cocoa GUI!
So I immediately opened XCode, generated a Cocoa application, and started messing around with Interface Builder. Here's what I came up with:
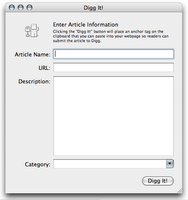
From Interface Builder I stubbed out my controller class (MVC after all!) and wired the button to an action method. I also decided to use Cocoa Bindings to wire the text fields to NSString properties in my model without any code. Now since my model only needed to expose four string properties (one for each field in my window) I chose to cheat a bit and just added the four properties to the controller.
Since Objective-C doesn't have automatic memory management, you typically manage the memory allocation and deallocation in the accessors. Fortunately Accessorizor came to my rescue and generated that code for me. So the only thing left for me to code was to pass my four text values to my Ruby script. A simple Google search resulted in this page on Cocoa Dev Central that describes how to call the 'ls' unix command from Cocoa. I quickly adapted it for calling my Ruby script. The one change I made was that I added my Ruby script to my XCode project as a resource so that it would be included in the application bundle at build time. So I added this line for finding it:
NSString *path = [[NSBundle mainBundle] pathForResource:@"digg" ofType:@"rb"];
Finally I decided it would be nice to just put the generated anchor tag on the clipboard when I click on the "Digg It" button. So I perused my "Cocoa Programming For Mac OS X" book and added these three lines:
NSPasteboard *pb = [NSPasteboard generalPasteboard];
[pb declareTypes:[NSArray arrayWithObject:NSStringPboardType] owner:self];
[pb setString:string forType:NSStringPboardType];
and voila:

2 comments:
Conveniently, your post is about Digg's three favorite topics: Digg itself, Apple, and Ruby. I wonder if anyone will bite?
I haven't done any Cocoa programming since Cocoa Bindings was introduced, but it sounds very handy (and a lot like using a CompoundPropertyModel with Wicket, which wasn't necessarily the first at doing anything but is what I'm most familiar with).
This is an ideal canidate for a TextMate snippet.
Post a Comment